Angular JS
2022. 4. 6. 11:12ㆍStudy/JavaScript
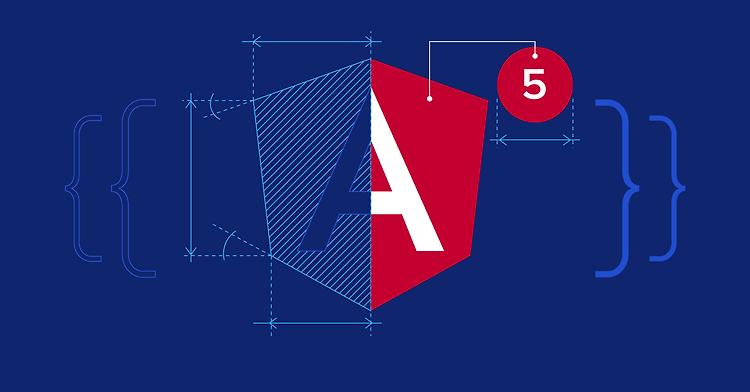
Angular JS란
https://www.w3schools.com/angular/default.asp
앵귤러JS는 자바스크립트 프레임워크들 중에서 최근 가장 주목 받는 프레임워크로 MVVM (Model View ViewModel) 패턴을 가장 잘 구현 하였다고 알려져 있다.
이를 이용하면 HTML기반에서 MVC(Model View Controller) 패턴을 적용하여 적은 소스 코드로 웹 어플리케이션을 쉽고 빠르게 만들 수 있다.
라이브러리가 아니고 프레임워크이므로 정해진 기본구조 위에 피룡한 기능을 갖다 얹기만 하면 된다.
즉, 건축을 하는 것이 아니고 지어진 건물을 구입하는 것이다.
현재 서버 측 스크립트는 프레임워크가 대세이고, 그 프레임워크가 바로 MVC (Model View Controller) 패턴이다.
이러한 서버 측 스크립트의 변화에 이어 클라이언트 측 스크립트에서도 새로운 변화의 바람이 불었따. 그때 등장한 것이 바로 앵귤러 JS 이다.
Angular란 무엇인가?
Angular JS란 동적인 웹앱을 구현하기 위해 구글에서 제작, 배포하고 있는 구조적 프레임워크라고 합니다. 특히 Angular의 데이타 바인딩(data binding)과 의존성주입(dependency injecion)은 코드를 매우 간결하게 만들어 줍니다. 일반적으로, 동적 어플리케이션과 정적인 문서 사이의 문제점은 다음과 같이 해결합니다.
* libery - 유용한 기능들을 모아 놓은 것을 말합니다. ex) jQuery
* frameworks - 웹 어플리케이션에 대한 특별한 완성본을 말합니다.
이런 상황에서 Angular는 좀 더 진보되고 directives라는 것을 이용해서 브라우저에게 새로운 문구를 알려줍니다.
데이타 바인딩은 {{}}로 표현합니다.
DOM 제어 구조는 반복과, 보여주기, DOM의 일정 부분을 숨길 수 있습니다.
폼과 폼 유효성 검사에 사용할 수 있습니다.
DOM 엘리먼트에 이벤트 핸들링과 같은 새로운 행동을 부여할 수 있습니다.
HTML을 그룹핑하여 재사용가능한 컴포넌트로 제작할 수 있습니다.
호환성 알아보기
최신 브라우저인 크롬, 파이어폭스, 사파리와 IE9-11 버전까지 지원 합니다.
앵귤러JS는 제이쿼리 라이브러리를 사용하고 있습니다. (jQLite)
Angular 장점
- 새로운 속성으로 HTML을 확장한다
- 단일 페이지 응용 프로그램(앱) 작업에 최적이다. (SPA, single page application)
- 쉽게 배울 수 있다.
Angular JS의 역사
Angular JS 1.0 버전은 2012년에 출시되었다.
2009년 구글 직원이었던 미스코 헤브리(Misko Hevery)는 Feedback 서비스를 개발하면서 자신이 만든 오픈 소스 라이브러리를 사용하여 17,000줄에 해당하는 UI 소스 코드를 단 3주만에 만들어 내는 파란을 일으켰다.
이 아이디어는 Angular JS 라는 오픈소스 커뮤니티가 만들어지는 시발점이 되었다.
cdn - js파일을 링크시켜 사용한다. (소형프로젝트에 적합)
npm - 다운받아 설치하여 사용한다. (웹팩같은 개념)
// ng-model ="변수 명" : 을 이용해 그룹화 해준다.
// value ="값" : 값을 지정해준다.(조건)
// ng-model의 변수에 value의 값이 저장된다.
// ng-switch로 영역을 만들어준다.
// ng-switch-when 으로 case를 만들어준다.
// ng-switch-when == ng-model 을 비교하게 되며, 쇼/하이드 해준다.
AngularJS를 사용하기 위해서 연결
cdn 혹은 npm 방법으로
경로에 유의하여 연결하도록 하자. (바인딩 후 오류가 날 수 있다)
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script src="./angular.min.js"></script>
</head>
EX1) 데이터 바인딩 ng-app, ng-model, ng-bind
Ajax를 사용하지 않아도, 쉽게 데이터 바인딩이 가능하다. (실시간 입,출력)
- ng-app : 앵귤러의 문법을 쓸 수 있는 범위 (여러개 있으면 안된다)
- ng-model : 입력
- ng-bind : ng-model에 입력한 값이 자동으로 출력된다. = {{}}
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
<p ng-bind="name"></p>
</div>
EX2) 초기값 ng-init
- ng-init : 초기값
<div ng-app="" ng-init="firstName='John'">
<p>The name is <span ng-bind="firstName"></span></p>
</div>
data-는 생략하고 사용할 수 있다.
<div data-ng-app="" data-ng-init="firstName='John'">
<p>The name is <span data-ng-bind="firstName"></span></p>
</div>
EX3) {{ }} == ng-bind
콧수염 연산자
출력하는 것, ng-bind와 같다. (출력하는 방법이 2가지 임)
연산하기
<div ng-app="">
<p>My first expression: {{ 5 + 5 }}</p>
</div>
input 값 바인딩
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
<p>YOUR NAME: {{name}}</p>
</div>
변수에 담아 CSS 제어
<div ng-app="" ng-init="myCol='red'">
<input style="background:{{myCol}}" ng-model="myCol" value="{{myCol}}">
</div>
변수에 담아 연산 가능
<div ng-app="" ng-init="quantity=1;cost=5">
<p>Total in dollar: {{ quantity * cost }}</p>
</div>
<!-- ng-bind로도 동일하게 사용 가능하다 -->
<div ng-app="" ng-init="quantity=1;cost=5">
<p>Total in dollar: <span ng-bind="quantity * cost"></span></p>
</div>
문자 출력
<div ng-app="" ng-init="firstName='John';lastName='Doe'">
<p>The name is {{ firstName + " " + lastName }}</p>
</div>
<!-- ng-bind로도 동일하게 사용 가능하다 -->
<div ng-app="" ng-init="firstName='John';lastName='Doe'">
<p>The name is <span ng-bind="firstName + ' ' + lastName"></span></p>
</div>
변수 출력 및 연산
<div ng-app="" ng-init="quantity=1;price=5">
Quantity: <input type="number" ng-model="quantity">
Costs: <input type="number" ng-model="price">
Total in dollar: {{ quantity * price }}
</div>
*객체 사용도 가능하다!!
<div ng-app="" ng-init="person={firstName:'John', lastName:'Doe'}">
<p>The name is {{ person.lastName + ' ' + person.firstName}}</p>
</div>
EX4) AngularJS Objects 객체사용
<div ng-app="" ng-init="person={firstName:'John',lastName:'Doe'}">
<p>The name is {{ person.lastName }}</p>
</div>
<div ng-app="" ng-init="person={firstName:'John',lastName:'Doe'}">
<p>The name is <span ng-bind="person.lastName"></span></p>
</div>
EX5) AngularJS Arrays 배열
<div ng-app="" ng-init="points=[1,15,19,2,40]">
<p>The third result is {{ points[2] }}</p>
</div>
<div ng-app="" ng-init="points=[1,15,19,2,40]">
<p>The third result is <span ng-bind="points[2]"></span></p>
</div>
EX6) AngularJS Module , AngularJS Controller ★☆★☆★
변수를 초기화해서 만들어놓는 문법, 객체를 모듈에 만들어놓는다.
AngularJS Module
var app = angular.module('myApp', []); // 이름, HTML ng-app에 연결
AngularJS Controller
app.controller('myCtrl', function($scope) { // 이름, HTML ng-controller에 연결
$scope.firstName= "John"; // 속성
$scope.lastName= "Doe";
});
예제:) 스크립트는 태그 밑에 넣는다.
<div ng-app="myApp" ng-controller="myCtrl">
First Name: <input type="text" ng-model="firstName"><br>
Last Name: <input type="text" ng-model="lastName"><br>
<br>
Full Name: {{firstName + " " + lastName}}
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.firstName= "John";
$scope.lastName= "Doe";
});
</script>
EX9) 반복(for) Repeating HTML Elements
ng-repeat = " 변수 in 배열명"
<div ng-app="" ng-init="names=['Jani','Hege','Kai']">
<ul>
<li ng-repeat="x in names">
{{ x }}
</li>
</ul>
</div>
객체배열, JSON형태
<div ng-app="" ng-init="names=[
{name:'Jani',country:'Norway'},
{name:'Hege',country:'Sweden'},
{name:'Kai',country:'Denmark'}]">
<ul>
<li ng-repeat="x in names">
{{ x.name + ', ' + x.country }}
</li>
</ul>
</div>
EX10) Create New Directives
<body ng-app="myApp">
<w3-test-directive></w3-test-directive>
<script>
var app = angular.module("myApp", []);
app.directive("w3TestDirective", function() {
return {
template : "<h1>Made by a directive!</h1>"
};
});
</script>
</body>
EX12) form - email 유효성 검사
이메일 유효성 검사를 쉽게 할 수있다.
valid | myForm.myAddress.$valid | 형식이 맞는지 체크 true, false 반환 |
dirty | myForm.myAddress.$dirty | 입력이 되었는지 체크 |
touched | myForm.myAddress.$touched | 터치 후 입력 했는지 체크 |
<form ng-app="" name="myForm" ng-init="myText = 'post@myweb.com'">
Email:
<input type="email" name="myAddress" ng-model="myText" required>
<p>Edit the e-mail address, and try to change the status.</p>
<h1>Status</h1>
<p>Valid: {{myForm.myAddress.$valid}} (if true, the value meets all criteria).</p>
<p>Dirty: {{myForm.myAddress.$dirty}} (if true, the value has been changed).</p>
<p>Touched: {{myForm.myAddress.$touched}} (if true, the field has been in focus).</p>
</form>
<AngularJS $http> ★☆★☆★
html을 따로 만들어 놓고 필요할때 load 한다.
html파일의 분리
AJAX의 원리와 같다.
EX13) html 가져오기
index.html
response.data = 문자열 형태로 가져온다
<div ng-app="myApp" ng-controller="myCtrl">
<h1>{{myWelcome}}</h1>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope, $http) {
$http.get("./welcome.html") // html파일 링크
.then(function(response) { // then : 접근
$scope.myWelcome = response.data;
});
});
</script>
welcome.html
Hello AngularJS Students
EX14)
<div ng-app="myApp" ng-controller="myCtrl">
<h1>{{myWelcome}}</h1>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope, $http) {
$http({
method : "GET", // or POST
url : "welcome.html"
}).then(function mySucces(response) { // mySucces 성공하면
$scope.myWelcome = response.data;
}, function myError(response) { // myError 실패하면
$scope.myWelcome = response.statusText; // 에러메세지가 자동으로 들어감
});
});
</script>
EX15) JSON ***** 객체 배열을 이용한 리스트 출력
response.data.records(이름)를 이용해 객체배열을 출력한다.
<div ng-app="myApp" ng-controller="customersCtrl">
<ul>
<li ng-repeat="x in myData">
{{ x.Name + ', ' + x.City + ', ' + x.Country }}
</li>
</ul>
</div>
<!--
<div ng-app="myApp" ng-controller="customersCtrl">
<table>
<tr ng-repeat="x in myData">
<td>{{ x.Name }}</td>
<td>{{ x.City }}</td>
<td>{{ x.Country }}</td>
</tr>
</table>
</div>
-->
<script>
var app = angular.module('myApp', []);
app.controller('customersCtrl', function($scope, $http) {
$http.get("customers.html").then(function (response) {
$scope.myData = response.data.records;
});
});
</script>
cusomers.html
JSON타입 형태, 객체배열
{
"records":[
{"Name":"Alfreds Futterkiste","City":"Berlin","Country":"Germany"},
{"Name":"Ana Trujillo Emparedados y helados","City":"México D.F.","Country":"Mexico"},
{"Name":"Antonio Moreno Taquería","City":"México D.F.","Country":"Mexico"},
{"Name":"Around the Horn","City":"London","Country":"UK"},
{"Name":"B's Beverages","City":"London","Country":"UK"},
{"Name":"Berglunds snabbköp","City":"Luleå","Country":"Sweden"},
{"Name":"Blauer See Delikatessen","City":"Mannheim","Country":"Germany"},
{"Name":"Blondel père et fils","City":"Strasbourg","Country":"France"},
{"Name":"Bólido Comidas preparadas","City":"Madrid","Country":"Spain"},
{"Name":"Bon app'","City":"Marseille","Country":"France"},
{"Name":"Bottom-Dollar Marketse","City":"Tsawassen","Country":"Canada"},
{"Name":"Cactus Comidas para llevar","City":"Buenos Aires","Country":"Argentina"},
{"Name":"Centro comercial Moctezuma","City":"México D.F.","Country":"Mexico"},
{"Name":"Chop-suey Chinese","City":"Bern","Country":"Switzerland"},
{"Name":"Comércio Mineiro","City":"São Paulo","Country":"Brazil"}
]
}
EX15-1)
<style>
table, th , td {border: 1px solid grey; border-collapse: collapse; padding: 5px;}
table tr:nth-child(odd) {background-color: #f1f1f1;}
table tr:nth-child(even) {background-color: #ffffff;}
</style>
<div ng-app="myApp" ng-controller="customersCtrl">
<table>
<tr ng-repeat="x in names">
<td>{{ x.Name }}</td>
<td>{{ x.Country }}</td>
</tr>
</table>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('customersCtrl', function($scope, $http) {
$http.get("customers.json")
.then(function (response) {
$scope.names = response.data.records;
});
});
</script>
EX15-2) 정렬하기 | orderBy, uppercase, $index
변수 in 배열이름 | orderBy : 'key/필드 값'
orderBy | orderBy : '기준' 오름차순 |
uppercase | 대문자 변경 |
$index | 인덱스 뽑아오기 |
<style>
table, th , td {border: 1px solid grey; border-collapse: collapse; padding: 5px;}
table tr:nth-child(odd) {background-color: #f1f1f1;}
table tr:nth-child(even) {background-color: #ffffff;}
</style>
<div ng-app="myApp" ng-controller="customersCtrl">
<table>
<tr ng-repeat="x in names | orderBy : 'Country'">
<td>{{ $index + 1 }}</td>
<td>{{ x.Name }}</td>
<td>{{ x.City }}</td>
<td>{{ x.Country | uppercase }}</td>
</tr>
</table>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('customersCtrl', function($scope, $http) {
$http.get("customers.json")
.then(function (response) {
$scope.names = response.data.records;
});
});
</script>
<AngularJS Select Boxes>
EX16) Creating a Select Box Using ng-options
selectbox의 옵션을 제어할 수 있다.
<div ng-app="myApp" ng-controller="myCtrl">
<select ng-model="selectedName" ng-options="x for x in names"></select>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.names = ["Emil", "Tobias", "Linus"];
});
</script>
EX16-1) ng-repeat을 이용해 selectbox 사용하기 (권장★)
<div ng-app="myApp" ng-controller="myCtrl">
<select>
<option ng-repeat="x in names">{{x}}</option>
</select>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.names = ["Emil", "Tobias", "Linus"];
});
</script>
EX16-2) selecbox 선택한 내용 출력하기
<div ng-app="myApp" ng-controller="myCtrl">
<p>Select a car:</p>
<select ng-model="selectedCar">
<option ng-repeat="x in cars" value="{{x.model}}">{{x.model}}</option>
</select>
<h1>You selected: {{selectedCar}}</h1>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.cars = [
{model : "Ford Mustang", color : "red"},
{model : "Fiat 500", color : "white"},
{model : "Volvo XC90", color : "black"}
];
});
</script>
EX16-3) selectbox와 연계된 정보 출력하기
select option 에서 선택한 값이
ng-model = "SelectedCar"에 전달되어
((selectedCar.model}} {{selectedCar.color}} 에 출력된다.
<div ng-app="myApp" ng-controller="myCtrl">
<p>Select a car:</p>
<select ng-model="selectedCar" ng-options="x.model for x in cars">
</select>
<h1>You selected: {{selectedCar.model}}</h1>
<p>Its color is: {{selectedCar.color}}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.cars = [
{model : "Ford Mustang", color : "red"},
{model : "Fiat 500", color : "white"},
{model : "Volvo XC90", color : "black"}
];
});
</script>
<AngularJS Events>
EX17) Mouse Events
ng-mouseenter
ng-mouseover
ng-mousemove
ng-mouseleave
ng-mousedown
ng-mouseup
ng-click
<div ng-app="myApp" ng-controller="myCtrl">
<h1 ng-mousemove="count = count + 1">Mouse Over Me!</h1>
<h2>{{ count }}</h2>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.count = 0;
});
</script>
EX17-1)
<div ng-app="myApp" ng-controller="myCtrl">
<button ng-click="count = count + 1">Click Me!</button>
<p>{{ count }}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.count = 0;
});
</script>
EX17-2) 함수를 사용한 *****
앱에 메소드 (함수)를 만들어 사용하기
myFunction() 함수를 호출한다.
$scope.myFunction = function(){...}
<div ng-app="myApp" ng-controller="myCtrl">
<button ng-click="myFunction()">Click Me!</button>
<p>{{ count }}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.count = 0;
$scope.myFunction = function() {
$scope.count++;
// this.count++;
}
});
</script>
EX17-3) Toggle, True/False, ng-show='true/false' ***
<div ng-app="">
<div>
<a href="" ng-click="toggle = !toggle" ng-init="toggle = true">Toggle nav</a>
<ul ng-show="toggle">
<li>Link 1</li>
<li>Link 2</li>
<li>Link 3</li>
</ul>
</div>
</div>
EX17-4) 함수를 사용해서 토글 구현하기
<div ng-app="myApp" ng-controller="myCtrl">
<button ng-click="myFunc()">Click Me!</button>
<div ng-show="showMe">
<h1>Menu:</h1>
<div>Pizza</div>
<div>Pasta</div>
<div>Pesce</div>
</div>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.showMe = false;
$scope.myFunc = function() {
$scope.showMe = !$scope.showMe;
}
});
</script>
<AngularJS Forms>
EX18) Radiobuttons
radio버튼을 이용해 컨텐츠 출력하기
<div ng-app="">
<form>
Pick a topic:
<input type="radio" ng-model="myVar" value="dogs">Dogs
<input type="radio" ng-model="myVar" value="tuts">Tutorials
<input type="radio" ng-model="myVar" value="cars">Cars
</form>
<div ng-switch="myVar">
<div ng-switch-when="dogs">
<h1>Dogs</h1>
<p>Welcome to a world of dogs.</p>
</div>
<div ng-switch-when="tuts">
<h1>Tutorials</h1>
<p>Learn from examples.</p>
</div>
<div ng-switch-when="cars">
<h1>Cars</h1>
<p>Read about cars.</p>
</div>
</div>
<p>The ng-switch directive hides and shows HTML sections depending on the value of the radio buttons.</p>
</div>
EX18-1) Selectbox
<div ng-app="">
<form>
Select a topic:
<select ng-model="myVar">
<option value="">
<option value="dogs">Dogs
<option value="tuts">Tutorials
<option value="cars">Cars
</select>
</form>
<div ng-switch="myVar">
<div ng-switch-when="dogs">
<h1>Dogs</h1>
<p>Welcome to a world of dogs.</p>
</div>
<div ng-switch-when="tuts">
<h1>Tutorials</h1>
<p>Learn from examples.</p>
</div>
<div ng-switch-when="cars">
<h1>Cars</h1>
<p>Read about cars.</p>
</div>
</div>
</div>
<AngularJS Form Validation>
$valid | (boolean) 정해진 규칙을 기반으로 맞는지 확인 |
$invalid | (boolean) 정해진 규칙을 기반으로 틀린지 확인 |
$dirty | (boolean) 입력된 값이 변경되었는지 확인 |
$error | 에러를 보여준다. |
EX19) E-mail 유효성검사
<div ng-app="">
<form name="myForm">
<input type="email" name="myInput" ng-model="myInput">
</form>
<h1>{{myForm.myInput.$valid}}</h1>
</div>
EX19-1) 필수항목 미 입력시 메시지 출력
<div ng-app="">
<form name="myForm">
<p>Name:
<input name="myName" ng-model="myName" required>
<span ng-show="myForm.myName.$touched && myForm.myName.$invalid">The name is required.</span>
</p>
<p>Adress:
<input name="myAddress" ng-model="myAddress" required>
</p>
</form>
</div>
EX19-2) 입력시 css변경
<style>
input.ng-invalid {background-color:pink;}
input.ng-valid {background-color:lightgreen;}
</style>
<div ng-app="">
<p>Try writing in the input field:</p>
<form name="myForm">
<input name="myName" ng-model="myName" required>
</form>
</div>
EX19-3) 입력 오류 메시지 출력
<h2>Validation Example</h2>
<style>
input.ng-invalid {background-color:pink;}
input.ng-valid {background-color:lightgreen;}
</style>
<form ng-app="myApp" ng-controller="validateCtrl"
name="myForm" novalidate>
<p>Username:<br>
<input type="text" name="user" ng-model="user" required>
<span style="color:red" ng-show="myForm.user.$dirty && myForm.user.$invalid">
<span ng-show="myForm.user.$error.required">Username is required.</span>
</span>
</p>
<p>Email:<br>
<input type="email" name="email" ng-model="email" required>
<span style="color:red" ng-show="myForm.email.$dirty && myForm.email.$invalid">
<span ng-show="myForm.email.$error.required">Email is required.</span>
<span ng-show="myForm.email.$error.email">Invalid email address.</span>
</span>
</p>
<p>
<input type="submit"
ng-disabled="myForm.user.$dirty && myForm.user.$invalid ||
myForm.email.$dirty && myForm.email.$invalid">
</p>
</form>
<script>
var app = angular.module('myApp', []);
app.controller('validateCtrl', function($scope) {
$scope.user = 'John Doe';
$scope.email = 'john.doe@gmail.com';
});
</script>
EX19-4) Create New Directives (콤포넌트)
- 콤포넌트 : 태그를 생성, 모듈화
- 모듈화 : 만들어놓고 가져다 쓰는 개념
- 바인딩 : 실시간으로 바꿈
- SPA : single page application의 기초개념(?)
var app = angular.module("myApp", []);
app.directive("해당태그", function(){
return {
template : "내용, 태그"
};
});
앵귤러 내 태그명을 카멜표기법으로 썼을때는
HTML태그는 하이픈 ( - ) 으로 표기해줘야 함을 유의하자~!
둘다 소문자로 표기했을 때는 문제가 없음!
<div ng-app="myApp">
<w3-test-directive></w3-test-directive>
<headers></headers>
<visuals></visuals>
<script>
var app = angular.module("myApp", []);
app.directive("w3TestDirective", function() {
return {
template : "<h1>Made by a directive!</h1>"
};
});
</script>
</div>
<div ng-app="myApp">
<headers></headers>
<visuals></visuals>
<contents></contents>
<footers></footers>
<script>
var app = angular.module("myApp", []);
app.directive("headers", function() {
return {
template : "<h1>Made by a directive!</h1>"
};
});
app.directive("visuals", function() {
return {
template : "<h2>Visual Area</h2>"
};
});
app.directive("contents", function() {
return {
template : "<h2>Contents Area</h2>"
};
});
app.directive("footers", function() {
return {
template : "<h2>Footer Area</h2>"
};
});
</script>
</div>
EX20) AngularJS Includes
큰 따옴표 안에 작은 따옴표를 꼭 써줘야 한다
ng-include = " ' ' "
<div ng-app="">
<style>
.wrap{width: 1200px; margin: 0 auto;}
header{background:green}
article{background:pink}
footer{background:orange}
</style>
<div class="wrap">
<div ng-include="'./component/header.html'"></div>
<div ng-include="'./component/contents.html'"></div>
<div ng-include="'./component/footer.html'"></div>
</div>
<!-- <div ng-include="'myFile.html'"></div> -->
</div>
<AngularJS Routing> 라우팅
*아래 스크립트 연결
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-route.js"></script>
EX21) 페이지 동적 호출
버전에 주의해야 한다.
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-route.js"></script>
<!-- EX21) 페이지 동적 호출 -->
<div ng-app="myApp">
<a href="#/">Main</a>
<a href="#red">Red</a>
<a href="#green">Green</a>
<a href="#blue">Blue</a>
<div ng-view></div>
</div>
<script>
var app = angular.module("myApp", ["ngRoute"]);
app.config(function($routeProvider) {
$routeProvider
.when("/", {
templateUrl : "main.html"
})
.when("/red", {
templateUrl : "red.html"
})
.when("/green", {
templateUrl : "green.html"
})
.when("/blue", {
templateUrl : "blue.html"
});
});
</script>
EX21-1) 페이지 동적 호출 2
<div ng-app="myApp">
<a href="#/">Main</a>
<a href="#banana">banana</a>
<a href="#tomato">tomato</a>
<div ng-view></div>
<script>
var app = angular.module("myApp", ["ngRoute"]);
app.config(function($routeProvider) {
$routeProvider
.when("/", {
template : "<h1>Main</h1><p>Click on the links to change this content</p>"
})
.when("/banana", {
template : "<h1>Banana</h1><p>Bananas contain around 75% water.</p>"
})
.when("/tomato", {
template : "<h1>Tomato</h1><p>Tomatoes contain around 95% water.</p>"
});
});
</script>
</div>
'Study > JavaScript' 카테고리의 다른 글
React JS (0) | 2022.04.15 |
---|---|
Vue JS (1) | 2022.04.08 |
마우스 휠 중복 과다 제어 막기 (0) | 2022.03.24 |
해상도별 이미지 교체 window resize (0) | 2022.01.11 |
클릭 시 Video 주소 바꾸기 (0) | 2021.12.23 |