해상도별 이미지 교체 window resize
2022. 1. 11. 10:37ㆍStudy/JavaScript
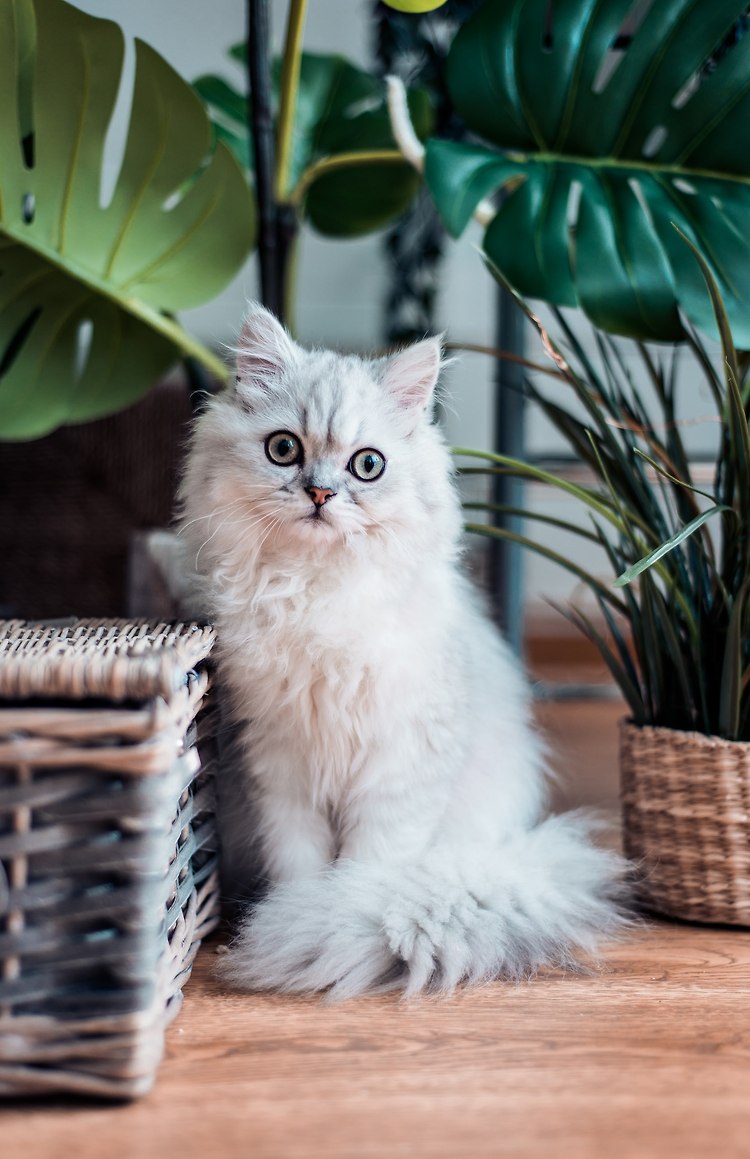
반응형 웹사이트 제작 시 해상도 처리 및 감지 방법에는 2가지가 있다.
- CSS의 미디어쿼리 사용
- Javascript의 $(window).width();와 if문 사용
CSS로는 미디어쿼리와 background 속성을 이용해 해상도별 이미지를 수정할 수 있지만 마크업을 수정할 순 없다.
이때 Javascript로 브라우저의 가로 값을 계산하여 해상도별로 마크업을 수정해줄 수 있다.
resize event
// jQuery
$(window).resize(function(){
// script
});
// JavaScript - addEventListener
window.addEventListener('resize', function(event) {
// script
}, true);
// JavaScript - onresize
window.onresize = function(event){
// script
};
예제:)
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.img1{width: 100%}
</style>
<script src="jquery-1.8.3.min.js"></script>
<script>
$(document).ready(function(){
var screenSize = $(window).width();
function screenW(){
if(screenSize>1280){
$('.img1').attr('src','a1.jpg');
}else if(screenSize>1024){
$('.img1').attr('src','a2.jpg');
}else if(screenSize>768){
$('.img1').attr('src','a3.jpg');
}else if(screenSize>640){
$('.img1').attr('src','a4.jpg');
}else{
$('.img1').attr('src','a5.jpg');
}
}
screenW(); //함수호출
$(window).resize(function(){
screenSize = $(window).width();
screenW();//함수호출
});
});
</script>
</head>
<body>
<div class="box">
<img class="img1" src="a1.jpg" alt="이미지">
</div>
</body>
</html>
참고:)
https://stackoverflow.com/questions/641857/javascript-window-resize-event
JavaScript window resize event
How can I hook into a browser window resize event? There's a jQuery way of listening for resize events but I would prefer not to bring it into my project for just this one requirement.
stackoverflow.com
세로값 맞추기 예제 - height();
기준이 되는 이미지등의 높이값을 스크립트로 받아와, 그 값을 넣어주는 방식이다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{margin: 0; padding: 0}
img{border: 0; vertical-align: top}
.box1{overflow: hidden; }
.box1 img{width: 100%;}
.box1 div{width: 48%; float: left; margin: 50px 1%; border:1px solid red; box-sizing: border-box}
.box2{overflow: hidden;}
.box2 img{width: 100%;}
.box2 div{float: left; margin: 50px 1%; border:1px solid red; box-sizing: border-box;}
.box2 div:nth-child(1){width:58%}
.box2 div:nth-child(2){width:38%}
</style>
<script src="jquery-1.7.1.min.js"></script>
<script>
$(document).ready(function() {
var boxHeight = $('.box2 div:eq(0)').height();
$(".box2 div:eq(1)").css('height',boxHeight);
$(window).resize(function(){
boxHeight = $('.box2 div:eq(0)').height();
$(".box2 div:eq(1)").css('height',boxHeight);
});
});
</script>
</head>
<body>
<div class="box1">
<div><img src="COBAIN.jpg" alt=""></div>
<div><img src="MAYER.jpg" alt=""></div>
</div>
<div class="box2">
<div><img src="COBAIN.jpg" alt=""></div>
<div>Lorem ipsum dolor sit amet consectetur adipisicing elit. Mollitia quaerat, corporis culpa dolores deserunt quasi perferendis laudantium molestiae ratione consequatur, suscipit libero nulla ut illo possimus, cumque perspiciatis veniam vero.</div>
</div>
</body>
</html>
세로값 맞추기 예제 - outerheight();
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{margin: 0; padding: 0}
img{border: 0; vertical-align: top}
.contactUs{margin: 0 5% 100px 5%; overflow: hidden; width: 90%}
.contactUs h3{text-align: center; margin-bottom: 50px}
.contactUs div{ border: 1px solid #ccc; float: left; box-sizing: border-box; box-shadow:3px 3px 3px #bbb; margin: 1% .5%; padding: 2%; width: 32%}
.contactUs h4{text-align: center; margin-bottom: 1.5%}
.contactUs address, p{text-align: center; font-family: Arial, Helvetica, sans-serif; line-height: 1.5em}
</style>
<script src="jquery-1.7.1.min.js"></script>
</head>
<body>
<div class="contactUs">
<div class="guest">
<h4>Guest Education Centre</h4>
<address>
Questions or concerns about products?<br>The GEC is here to help.<br><br>
text us on your mobile: 1.877.263.9300<br><br>
phone: 1.877.263.9300<br>phone: 1.604.215.9300<br><br>
Weekdays 5am-9pm<br>Weekends 6am-6pm<br><br>
1380 Burrard Street, Suite 200<br>Vancouver, BC<br>Canada<br>V6Z 2H3
</address>
</div>
<div class="store">
<h4>Store Support Centre</h4>
<address>
Our Store Support Centre (SSC) is home to everyone from business operations to community relations.<br><br>
Contact the SSC at:<br>1818 Cornwall Avenue<br>Vancouver, BC<br>Canada<br>V6J 1C7<br><br>
phone: 1.604.732.6124<br>fax: 1.604.874.6124
</address>
</div>
<div class="email">
<h4>Email</h4>
<p>For answers to general questions about lululemon athletica, please email the Guest Education Centre. If you're looking to speak with someone quickly, you can text or call the GEC at 1.877.263.9300 or try out our live chat (available Weekdays 5am-9pm, Weekends 6am-6pm).</p>
</div>
<div class="press">
<h4>Press Inquiries</h4>
<p>Members of the media can email our Public Relations Department.<br><br>am - 10pm PST weekdays<br>6am - 6pm PST weekends</p>
</div>
<div class="chat">
<h4>Live Chat</h4>
<p>online chat<br><br>Weekdays 5am-9pm<br>Weekends 6am-6pm</p>
</div>
<div class="sales">
<h4>Strategic Sales</h4>
<address>Please email us or call 1.877.263.9300.<br><br>You can also read more about our Strategic Sales program here.</address>
</div>
</div>
<script>
$(document).ready(function() {
var boxHeight2 = $('.contactUs div:eq(0)').outerHeight();
for(var i=0; i<6; i++){
$(".contactUs div:eq("+ i +")").css('height',boxHeight2);
}
$(window).resize(function(){
$('.contactUs .guest').css('height','auto');
boxHeight2 = $('.contactUs div:eq(0)').outerHeight();
for(var i=0; i<6; i++){
$(".contactUs div:eq("+ i +")").css('height',boxHeight2);
}
});
});
</script>
</body>
</html>
'Study > JavaScript' 카테고리의 다른 글
Angular JS (0) | 2022.04.06 |
---|---|
마우스 휠 중복 과다 제어 막기 (0) | 2022.03.24 |
클릭 시 Video 주소 바꾸기 (0) | 2021.12.23 |
링크 시 매개변수(parameter)파라미터 값 넘기기 (0) | 2021.12.21 |
Mobile 홈 화면 전체 화면 모드 (0) | 2021.12.21 |